· Python · 2 min read
How to access the index in a Python For Loop
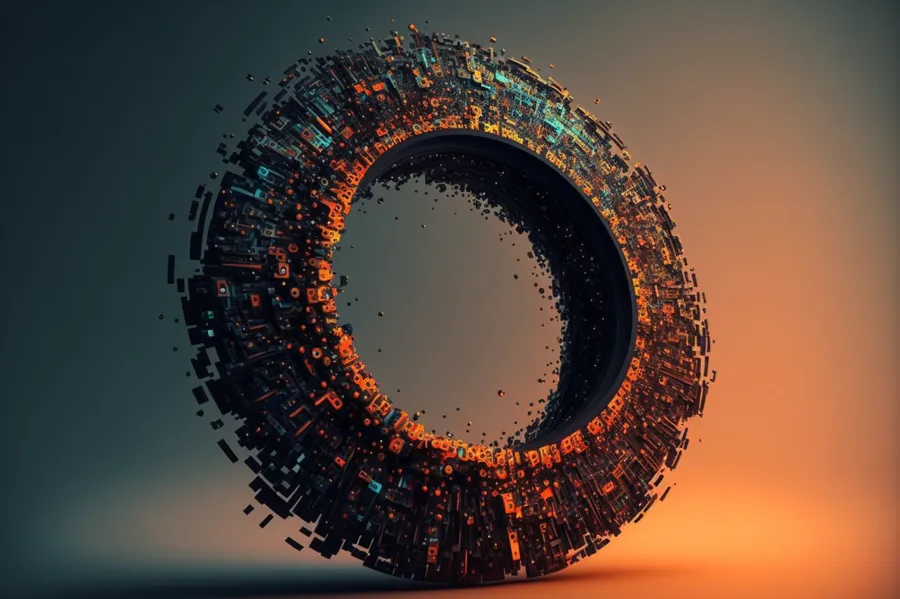
For Loops
A for loop is a control flow structure that allows you to iterate over a sequence of elements, such as a list or a string. The basic syntax of a for loop in Python is
for element in sequence:
# code to be executed on each iteration
Sequence is the name of the thing you want to iterate over, and element is a variable that takes on the value of each element in the sequence, one at a time.
Here is an example of a for loop that iterates over a list of integers and prints the square of each number.
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number ** 2)
Unlike some programming languages, python for loops do not have an accompanying index
What is the index
In a sequence such as a list or a string, an index is an integer value that refers to a specific element in the sequence. Indices are used to access and manipulate the elements of a sequence.
In Python, sequences are indexed starting from 0, so the first element of a sequence has an index of 0, the second element has an index of 1, and so on.
How to access the index in a for loop with Python
We’re going to use the enumerate
function.
The enumerate() function is a built-in function in Python that takes an iterable object (such as a list or a string) and returns an iterator that produces tuples. Each tuple contains an index and the corresponding element from the input object. Here is an example of how to use enumerate() in a for loop.
Here is a code example of how to do this.
fruits = ['apple', 'banana', 'cherry', 'date']
for i, fruit in enumerate(fruits):
print(f'Index {i}: {fruit}')
# Output
# Index 0: apple
# Index 1: banana
# Index 2: cherry
# Index 3: date
In this ultimate guide, you will learn how to use Pandas to perform various data manipulation tasks, such as cleaning, filtering, sorting and aggregating data.